- Status
- Offline
- Joined
- Oct 22, 2024
- Messages
- 153
- Reaction score
- 81
Hey everyone! Today I’m sharing an open-source color triggerbot for Valorant written in Python using PyQt5 for a smooth interface. The project is abandoned by the author but fully functional — a perfect base for customization. Important: it’s an external cheat, which reduces detection risks, but always test it on accs you don’t mind losing! Author: DavidUmm
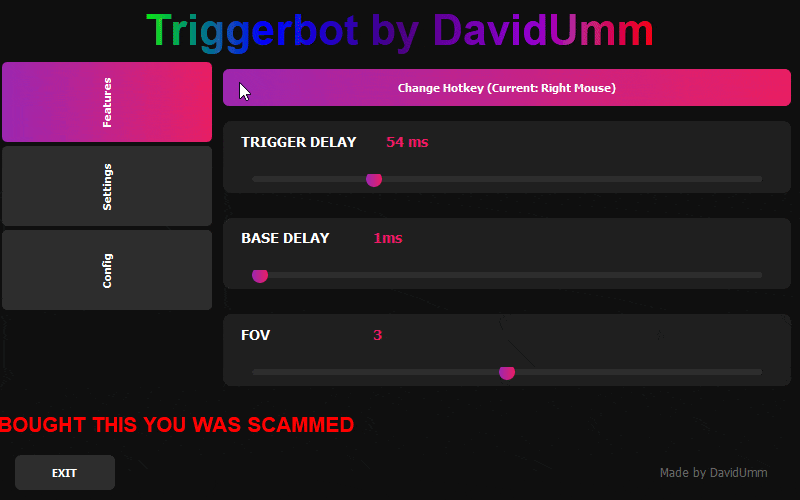
What’s in this source?
- Color trigger
Allows adaptation to the environment: for example, on Bind map, red (255, 0, 0) is effective due to contrast with the sandy background.
For camouflage skins, manual adjustment of values in line 352 of this source is required.
- Settings
Delays: base (up to 200 ms) + random (10–200 ms) to mimic human reaction.
Keys: configurable via the interface (default aim is bound to the mouse side button).
- Control interface
Usage example: separate presets for Icebox (FOV=4) and Split (blue shades).
What dependencies need to be installed?
Before launching, install these Python dependencies via the command:pip install PyQt5 numpy dxcam keyboard pywin32 win32api ctypes
Other modules like «threading», «json», etc., come bundled with Python upon installation.
Cheat installation guide
1. Install Python version 3.10 or higher. Pay attention to the version!2. Save the sources into files «gui.py» and «triggerbot.py»:
Python:
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QWidget, QVBoxLayout, QHBoxLayout,
QLabel, QSlider, QStyleFactory, QPushButton, QTabWidget, QDialog)
from PyQt5.QtCore import Qt, QTimer, QPropertyAnimation, QEasingCurve, QPoint
from PyQt5.QtGui import QFont, QColor, QPalette, QLinearGradient, QPainter, QPen
from triggerbot import Triggerbot
import win32api
class RainbowLabel(QLabel):
def [B]init[/B](self, text, parent=None):
super().[B]init[/B](text, parent)
self.setFixedHeight(60)
self.setFont(QFont('Arial', 32, QFont.Bold))
self.setAlignment(Qt.AlignCenter)
self.colors = [
QColor(255, 0, 0),
QColor(255, 127, 0),
QColor(255, 255, 0),
QColor(0, 255, 0),
QColor(0, 0, 255),
QColor(75, 0, 130),
QColor(148, 0, 211)
]
self.timer = QTimer(self)
self.timer.timeout.connect(self.update_colors)
self.timer.start(100)
self.offset = 0
def update_colors(self):
self.offset = (self.offset + 1) % len(self.colors)
self.update()
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
painter.fillRect(self.rect(), QColor("#0f0f0f"))
gradient = QLinearGradient(0, 0, self.width(), 0)
for i in range(len(self.colors)):
idx = (i + self.offset) % len(self.colors)
gradient.setColorAt(i / (len(self.colors) - 1), self.colors[idx])
painter.setPen(QPen(gradient, 1))
painter.setFont(self.font())
painter.drawText(self.rect(), Qt.AlignCenter, self.text())
class WarningLabel(QLabel):
def [B]init[/B](self, text, parent=None):
super().[B]init[/B](text, parent)
self.setFixedHeight(40)
self.setFixedWidth(800)
self.setFont(QFont('Arial', 16, QFont.Bold))
self.setStyleSheet("""
color: #ff0000;
background-color: #0f0f0f;
""")
self.pos_x = -self.width()
self.move_timer = QTimer(self)
self.move_timer.timeout.connect(self.update_position)
self.move_timer.start(16)
def update_position(self):
self.pos_x += 2
if self.pos_x > self.parent().width():
self.pos_x = -self.width()
self.move(self.pos_x, self.y())
class MainWindow(QMainWindow):
def [B]init[/B](self):
super().[B]init[/B]()
self.setWindowTitle('Triggerbot by DavidUmm')
self.setWindowFlags(Qt.FramelessWindowHint | Qt.WindowStaysOnTopHint)
self.setAttribute(Qt.WA_TranslucentBackground)
self.oldPos = None
if not self.verify_ownership():
print("Verification failed - Invalid version")
sys.exit()
self.triggerbot = Triggerbot()
self.init_ui()
self.triggerbot.initialize()
self.waiting_for_key = False
self.key_listen_timer = None
def verify_ownership(self):
return "DavidUmm" in self.windowTitle() or "DavidUmm" in [B]file[/B]
def init_ui(self):
self.setFixedSize(800, 500)
self.setStyleSheet("""
QMainWindow {
background-color: #0f0f0f;
border-radius: 20px;
}
QWidget#centralWidget {
background-color: #0f0f0f;
border-radius: 20px;
}
QWidget {
background-color: #0f0f0f;
color: #ffffff;
}
QTabWidget::pane {
border: none;
background-color: #1a1a1a;
border-radius: 10px;
}
QTabBar::tab {
background-color: #2d2d2d;
color: #ffffff;
padding: 15px 30px;
margin: 2px;
border-radius: 5px;
font-weight: bold;
min-width: 150px;
text-align: center;
}
QTabBar::tab:selected {
background: qlineargradient(x1:0, y1:0, x2:1, y2:0,
stop:0 #9c27b0, stop:1 #e91e63);
}
QTabBar::tab:vertical {
min-height: 50px;
max-height: 50px;
}
QSlider::groove:horizontal {
border: none;
height: 6px;
background: #2d2d2d;
border-radius: 3px;
}
QSlider::handle:horizontal {
background: qlineargradient(x1:0, y1:0, x2:1, y2:0,
stop:0 #9c27b0, stop:1 #e91e63);
width: 16px;
height: 16px;
margin: -5px 0;
border-radius: 8px;
}
QPushButton {
background-color: #2d2d2d;
color: white;
border: none;
padding: 12px;
border-radius: 6px;
font-weight: bold;
}
QPushButton:hover {
background: qlineargradient(x1:0, y1:0, x2:1, y2:0,
stop:0 #9c27b0, stop:1 #e91e63);
}
""")
main_widget = QWidget()
main_widget.setObjectName("centralWidget")
self.setCentralWidget(main_widget)
main_layout = QVBoxLayout(main_widget)
main_layout.setSpacing(0)
main_layout.setContentsMargins(0, 0, 0, 0)
title_label = RainbowLabel('Triggerbot by DavidUmm')
title_label.setAlignment(Qt.AlignCenter)
main_layout.addWidget(title_label)
tabs = QTabWidget()
tabs.setTabPosition(QTabWidget.West)
tabs.setStyleSheet("""
QTabWidget::pane {
border: none;
background-color: #1a1a1a;
border-radius: 10px;
}
QTabBar::tab {
background-color: #2d2d2d;
color: #ffffff;
padding: 15px 30px;
margin: 2px;
border-radius: 5px;
font-weight: bold;
min-width: 150px;
text-align: center;
}
QTabBar::tab:selected {
background: qlineargradient(x1:0, y1:0, x2:1, y2:0,
stop:0 #9c27b0, stop:1 #e91e63);
}
QTabBar::tab:vertical {
min-height: 50px;
max-height: 50px;
}
""")
content_widget = QWidget()
content_layout = QVBoxLayout(content_widget)
content_layout.setSpacing(20)
content_layout.setContentsMargins(30, 30, 30, 30)
self.status_label = QLabel('INACTIVE')
self.status_label.setAlignment(Qt.AlignCenter)
self.status_label.setStyleSheet("""
background-color: #1f1f1f;
color: #ff4444;
font-size: 20px;
font-weight: bold;
border-radius: 8px;
padding: 20px;
""")
content_layout.addWidget(self.status_label)
features_widget = QWidget()
features_layout = QVBoxLayout(features_widget)
features_layout.setSpacing(15)
def get_key_name(key_code):
mouse_buttons = {
0x01: "Left Mouse",
0x02: "Right Mouse",
0x04: "Middle Mouse",
0x05: "Mouse 4",
0x06: "Mouse 5"
}
return mouse_buttons.get(key_code, chr(key_code))
current_key_name = get_key_name(self.triggerbot.trigger_hotkey)
self.hotkey_btn = QPushButton(f'Change Hotkey (Current: {current_key_name})')
self.hotkey_btn.clicked.connect(self.start_key_capture)
features_layout.addWidget(self.hotkey_btn)
for name, range_min, range_max, default, suffix in [
('TRIGGER DELAY', 10, 200, self.triggerbot.trigger_delay, 'ms'),
('BASE DELAY', 1, 200, int(self.triggerbot.base_delay * 1000), 'ms'),
('FOV', 1, 5, self.triggerbot.pixel_fov, '')
]:
frame = QWidget()
frame.setStyleSheet("""
background-color: #1f1f1f;
padding: 20px;
border-radius: 8px;
margin-bottom: 10px;
""")
frame_layout = QVBoxLayout(frame)
frame_layout.setSpacing(12)
header_layout = QHBoxLayout()
header_layout.setContentsMargins(0, 0, 0, 0)
label = QLabel(name)
label.setStyleSheet("""
font-size: 14px;
font-weight: bold;
color: white;
padding: 5px;
""")
label.setMinimumWidth(120)
value_label = QLabel(f"{default}{suffix}")
value_label.setStyleSheet("""
color: #e91e63;
font-size: 14px;
font-weight: bold;
padding: 5px;
""")
value_label.setMinimumWidth(80)
header_layout.addWidget(label)
header_layout.addWidget(value_label)
header_layout.addStretch()
frame_layout.addLayout(header_layout)
slider = QSlider(Qt.Horizontal)
slider.setFixedHeight(20)
slider.setRange(range_min, range_max)
slider.setValue(default)
slider.setProperty('name', name)
frame_layout.addWidget(slider)
features_layout.addWidget(frame)
if name == 'TRIGGER DELAY':
slider.valueChanged.connect(lambda v, l=value_label: self.update_trigger_delay(v, l))
elif name == 'BASE DELAY':
slider.valueChanged.connect(lambda v, l=value_label: self.update_base_delay(v, l))
else:
slider.valueChanged.connect(lambda v, l=value_label: self.update_pixel_fov(v, l))
features_layout.addStretch()
tabs.addTab(features_widget, "Features")
settings_widget = QWidget()
settings_layout = QVBoxLayout(settings_widget)
color_frame = QWidget()
color_frame.setStyleSheet("""
background-color: #1f1f1f;
padding: 25px;
border-radius: 8px;
""")
color_layout = QVBoxLayout(color_frame)
color_header = QHBoxLayout()
color_label = QLabel("OUTLINE COLOR")
color_label.setStyleSheet("""
font-size: 16px;
font-weight: bold;
color: white;
""")
self.current_color_display = QLabel(f"CURRENT: {self.triggerbot.current_color}")
current_rgb = (self.triggerbot.R, self.triggerbot.G, self.triggerbot.B)
self.current_color_display.setStyleSheet(f"""
background-color: rgb{current_rgb};
color: black;
font-size: 14px;
font-weight: bold;
padding: 5px 15px;
border-radius: 4px;
""")
color_header.addWidget(color_label)
color_header.addWidget(self.current_color_display)
color_header.addStretch()
color_layout.addLayout(color_header)
buttons_layout = QHBoxLayout()
buttons_layout.setSpacing(20)
buttons_layout.setContentsMargins(0, 20, 0, 0)
#=======================================================================
# ______ _____ _____ _______ _____ [B]__ _ [B][/B] [B][I][/B] __[/I][/B]
# | [B][B]| _ \_ [I]|[/B] [B]| / [I][/I][/B]/ [I][/I] \| | / [I][/I] \| [I][/I] \ / _[/I][/B]|
# | |__ | | | || | | | | | | | | | | | | | | |[B]) | (_[/B]
# | [B]| | | | || | | | | | | | | | | | | | | _ / \_[/B] \
# | |[B][B]| |[/B]| || |_ | | | |[B][I]| |[/B]| | |[B][/I]| |[/B]| | | \ \ __[/B]) |
# |______|_____/_____| |[I]| \[B]__[/I]\[B][B]/|[/B][/B][/B]\[B][B]/|[I]| \[/I]\_[/B][/B]/
#
#=======================================================================
#
# EDIT THESE RGB VALUES TO CHANGE OUTLINE DETECTION COLORS
#
color_configs = [
("PURPLE", (250, 100, 250)), # Default Purple outline
("RED", (255, 50, 50)), # Red outline
("YELLOW", (250, 250, 40)) # Yellow outline
]
#
# When a color is selected, detection ranges are automatically set to:
# Red/Green/Blue + or - 25/20/20 for upper/lower thresholds
#
#=======================================================================
for color_name, rgb in color_configs:
btn = QPushButton(color_name)
btn.setFixedSize(150, 60)
btn.setProperty('rgb_values', rgb)
btn.setProperty('color_name', color_name)
btn.setStyleSheet(f"""
QPushButton {{
background-color: rgb{rgb};
color: black;
border: none;
border-radius: 8px;
font-weight: bold;
font-size: 16px;
margin: 5px;
}}
QPushButton:hover {{
background-color: rgb{tuple(min(255, c + 30) for c in rgb)};
}}
QPushButton:pressed {{
background-color: rgb{tuple(max(0, c - 30) for c in rgb)};
}}
""")
btn.clicked.connect(self.handle_color_button)
buttons_layout.addWidget(btn)
color_layout.addLayout(buttons_layout)
settings_layout.addWidget(color_frame)
settings_layout.addStretch()
tabs.addTab(settings_widget, "Settings")
config_widget = QWidget()
config_layout = QVBoxLayout(config_widget)
config_layout.setSpacing(20)
config_frame = QWidget()
config_frame.setStyleSheet("""
background-color: #1f1f1f;
padding: 25px;
border-radius: 8px;
""")
config_layout_inner = QVBoxLayout(config_frame)
config_layout_inner.setSpacing(30)
# Config Header
config_header = QLabel("CONFIG SETTINGS")
config_header.setStyleSheet("""
font-size: 20px;
font-weight: bold;
color: white;
margin-bottom: 20px;
""")
config_header.setAlignment(Qt.AlignCenter)
config_layout_inner.addWidget(config_header)
button_layout = QHBoxLayout()
button_layout.setSpacing(40)
save_btn = QPushButton("Save")
save_btn.setFixedSize(120, 35)
save_btn.setStyleSheet("""
QPushButton {
background: qlineargradient(x1: 0, y1: 0, x2: 1, y2: 0,
stop: 0 #9c27b0, stop: 1 #e91e63);
color: white;
border: none;
border-radius: 4px;
font-weight: bold;
font-size: 13px;
}
QPushButton:hover {
background: qlineargradient(x1: 0, y1: 0, x2: 1, y2: 0,
stop: 0 #7b1fa2, stop: 1 #c2185b);
}
""")
save_btn.clicked.connect(self.save_config)
reset_btn = QPushButton("Reset")
reset_btn.setFixedSize(120, 35)
reset_btn.setStyleSheet("""
QPushButton {
background-color: #f44336;
color: white;
border: none;
border-radius: 4px;
font-weight: bold;
font-size: 13px;
}
QPushButton:hover {
background-color: #d32f2f;
}
""")
reset_btn.clicked.connect(self.reset_config)
button_layout.addWidget(save_btn)
button_layout.addWidget(reset_btn)
button_layout.setAlignment(Qt.AlignCenter)
config_layout_inner.addLayout(button_layout)
config_layout.addWidget(config_frame)
config_layout.addStretch()
tabs.addTab(config_widget, "Config")
credits = QLabel('Made by DavidUmm')
credits.setStyleSheet("""
QLabel {
color: #666666;
font-size: 12px;
padding: 10px 15px;
}
""")
credits.setAlignment(Qt.AlignRight)
content_layout.addWidget(credits)
bottom_section = QWidget()
bottom_layout = QVBoxLayout(bottom_section)
bottom_layout.setSpacing(0)
bottom_layout.setContentsMargins(0, 0, 0, 0)
warning_label = WarningLabel('IF YOU BOUGHT THIS YOU WAS SCAMMED')
bottom_layout.addWidget(warning_label)
button_bar = QWidget()
button_bar_layout = QHBoxLayout(button_bar)
button_bar_layout.setContentsMargins(15, 10, 15, 10)
exit_btn = QPushButton("EXIT")
exit_btn.setFixedSize(100, 35)
exit_btn.setStyleSheet("""
QPushButton {
background-color: #1f1f1f;
color: #666666;
border: none;
border-radius: 4px;
font-size: 13px;
font-weight: bold;
padding: 0 15px; # Added horizontal padding
}
QPushButton:hover {
background-color: #f44336;
color: white;
}
""")
exit_btn.clicked.connect(self.close)
button_bar_layout.addWidget(exit_btn)
button_bar_layout.addStretch()
button_bar_layout.addWidget(credits)
bottom_layout.addWidget(button_bar)
main_layout.addWidget(tabs, 1)
main_layout.addWidget(bottom_section)
self.status_timer = self.startTimer(100)
def toggle_feature(self, feature, button):
if feature == 'humanization':
self.triggerbot.humanization = not self.triggerbot.humanization
button.setText('HUMANIZATION: ON' if self.triggerbot.humanization else 'HUMANIZATION: OFF')
elif feature == 'sticky_aim':
self.triggerbot.sticky_aim = not self.triggerbot.sticky_aim
button.setText('STICKY AIM: ON' if self.triggerbot.sticky_aim else 'STICKY AIM: OFF')
self.triggerbot.save_config()
def start_key_capture(self):
self.waiting_for_key = True
self.hotkey_btn.setText('Press any key or mouse button...')
self.hotkey_btn.setEnabled(False)
self.key_listen_timer = QTimer()
self.key_listen_timer.timeout.connect(self.check_mouse_input)
self.key_listen_timer.start(100)
def check_mouse_input(self):
if self.waiting_for_key:
mouse_buttons = {
0x01: "Left Mouse",
0x02: "Right Mouse",
0x04: "Middle Mouse",
0x05: "Mouse 4",
0x06: "Mouse 5"
}
for code, name in mouse_buttons.items():
if win32api.GetAsyncKeyState(code) < 0:
self.handle_new_hotkey(code, name)
return
def handle_new_hotkey(self, key_code, key_name):
self.triggerbot.trigger_hotkey = key_code
self.waiting_for_key = False
self.hotkey_btn.setEnabled(True)
self.hotkey_btn.setText(f'Change Hotkey (Current: {key_name})')
self.triggerbot.save_config()
if self.key_listen_timer:
self.key_listen_timer.stop()
def keyPressEvent(self, event):
if self.waiting_for_key:
key_text = event.text().upper()
if key_text:
self.handle_new_hotkey(event.nativeVirtualKey(), key_text)
def timerEvent(self, event):
if not hasattr(self, 'status_timer') or event.timerId() != self.status_timer:
return
try:
if win32api.GetAsyncKeyState(self.triggerbot.trigger_hotkey) < 0 or self.triggerbot.always_enabled:
self.status_label.setText('ACTIVE')
self.status_label.setStyleSheet("""
QLabel {
color: #44ff44;
font-size: 20px;
font-weight: bold;
}
""")
else:
self.status_label.setText('INACTIVE')
self.status_label.setStyleSheet("""
QLabel {
color: #ff4444;
font-size: 20px;
font-weight: bold;
}
""")
except:
pass
def update_trigger_delay(self, value, label):
self.triggerbot.trigger_delay = value
label.setText(f'{value} ms')
self.triggerbot.save_config()
def update_base_delay(self, value, label):
delay = value / 1000.0
self.triggerbot.base_delay = delay
label.setText(f'{value} ms')
self.triggerbot.save_config()
def update_pixel_fov(self, value, label):
self.triggerbot.pixel_fov = value
label.setText(f'FOV: {value}')
self.triggerbot.update_grab_zone()
self.triggerbot.save_config()
def handle_color_button(self):
btn = self.sender()
rgb = btn.property('rgb_values')
color_name = btn.property('color_name')
self.current_color_display.setText(f"CURRENT: {color_name}")
self.current_color_display.setStyleSheet(f"""
background-color: rgb{rgb};
color: black;
font-size: 14px;
font-weight: bold;
padding: 5px 15px;
border-radius: 4px;
""")
self.triggerbot.current_color = color_name
self.triggerbot.R = rgb[0]
self.triggerbot.G = rgb[1]
self.triggerbot.B = rgb[2]
self.triggerbot.R_high = rgb[0] + 25
self.triggerbot.R_low = rgb[0] - 25
self.triggerbot.G_high = rgb[1] + 20
self.triggerbot.G_low = rgb[1] - 20
self.triggerbot.B_high = rgb[2] + 20
self.triggerbot.B_low = rgb[2] - 20
self.triggerbot.save_config()
def closeEvent(self, event):
try:
self.status_timer = None
self.triggerbot.cleanup()
except:
pass
event.accept()
def save_config(self):
self.triggerbot.save_config()
self.show_notification("Config Saved!")
def reset_config(self):
self.triggerbot.base_delay = 0.001
self.triggerbot.trigger_delay = 50
self.triggerbot.pixel_fov = 3
self.triggerbot.current_color = "PURPLE"
self.triggerbot.R, self.triggerbot.G, self.triggerbot.B = 250, 100, 250
self.triggerbot.save_config()
self.update_all_displays()
self.show_notification("Config Reset!")
def show_notification(self, message):
popup = QDialog(self)
popup.setWindowFlags(Qt.FramelessWindowHint | Qt.Popup)
popup.setStyleSheet("""
QDialog {
background: qlineargradient(x1:0, y1:0, x2:1, y2:0,
stop:0 #9c27b0, stop:1 #e91e63);
border-radius: 10px;
}
""")
layout = QVBoxLayout(popup)
label = QLabel(message)
label.setStyleSheet("""
color: white;
padding: 20px 40px;
font-size: 16px;
font-weight: bold;
""")
label.setAlignment(Qt.AlignCenter)
layout.addWidget(label)
popup.setFixedSize(300, 100)
x = self.x() + (self.width() - popup.width()) // 2
y = self.y() + (self.height() - popup.height()) // 2
popup.move(x, y)
popup.show()
QTimer.singleShot(2000, popup.close)
def update_all_displays(self):
for slider in self.findChildren(QSlider):
if slider.property('name') == 'TRIGGER DELAY':
slider.setValue(self.triggerbot.trigger_delay)
elif slider.property('name') == 'BASE DELAY':
slider.setValue(int(self.triggerbot.base_delay * 1000))
elif slider.property('name') == 'FOV':
slider.setValue(self.triggerbot.pixel_fov)
current_rgb = (self.triggerbot.R, self.triggerbot.G, self.triggerbot.B)
self.current_color_display.setText(f"CURRENT: {self.triggerbot.current_color}")
self.current_color_display.setStyleSheet(f"""
background-color: rgb{current_rgb};
color: black;
font-size: 14px;
font-weight: bold;
padding: 5px 15px;
border-radius: 4px;
""")
current_key_name = {
0x01: "Left Mouse",
0x02: "Right Mouse",
0x04: "Middle Mouse",
0x05: "Mouse 4",
0x06: "Mouse 5"
}.get(self.triggerbot.trigger_hotkey, chr(self.triggerbot.trigger_hotkey))
self.hotkey_btn.setText(f'Change Hotkey (Current: {current_key_name})')
def mousePressEvent(self, event):
self.oldPos = event.globalPos()
def mouseMoveEvent(self, event):
if self.oldPos:
delta = event.globalPos() - self.oldPos
self.move(self.pos() + delta)
self.oldPos = event.globalPos()
def mouseReleaseEvent(self, event):
self.oldPos = None
if [B]name[/B] == '[B]main[/B]':
app = QApplication(sys.argv)
app.setStyle(QStyleFactory.create('Fusion'))
window = MainWindow()
window.show()
sys.exit(app.exec_())
Python:
import json
import time
import threading
import sys
import win32.win32api as win32api
from ctypes import WinDLL, windll
import numpy as np
import dxcam
import keyboard
import multiprocessing
from multiprocessing import Pipe, Process
user32, kernel32, shcore = (
WinDLL("user32", use_last_error=True),
WinDLL("kernel32", use_last_error=True),
WinDLL("shcore", use_last_error=True),
)
shcore.SetProcessDpiAwareness(2)
WIDTH, HEIGHT = [user32.GetSystemMetrics(0), user32.GetSystemMetrics(1)]
def calculate_grab_zone(pixel_fov):
ZONE = max(1, min(5, pixel_fov))
return (
int(WIDTH / 2 - ZONE),
int(HEIGHT / 2 - ZONE),
int(WIDTH / 2 + ZONE),
int(HEIGHT / 2 + ZONE),
)
class Triggerbot:
def [B]init[/B](self):
self.sct = None
self.triggerbot = False
self.exit_program = False
self.paused = False
self.initialized = False
self.auto_counter_strafe = False
self.humanization = False
self.sticky_aim = False
self.sticky_aim_thread = None
self.sticky_aim_stop_event = threading.Event()
self.parent_conn, self.child_conn = Pipe()
self.key_process = Process(target=self.key_worker, args=(self.child_conn,))
self.key_process.daemon = True
self.key_process.start()
self.current_color = "PURPLE"
self.R = 250
self.G = 100
self.B = 250
self.R_high = self.R + 25
self.R_low = self.R - 25
self.G_high = self.G + 20
self.G_low = self.G - 20
self.B_high = self.B + 20
self.B_low = self.B - 20
self.load_config()
self.update_grab_zone()
def load_config(self):
try:
with open('config.json', 'r') as json_file:
config = json.load(json_file)
self.trigger_hotkey = int(config.get('trigger_hotkey', '0x02'), 16)
self.always_enabled = config.get('always_enabled', False)
self.trigger_delay = config.get('trigger_delay', 50)
self.base_delay = config.get('base_delay', 0.001)
self.pixel_fov = config.get('pixel_fov', 3)
self.humanization = config.get('humanization', False)
self.sticky_aim = config.get('sticky_aim', False)
self.current_color = config.get('current_color', "PURPLE")
self.R = config.get('R', 250)
self.G = config.get('G', 100)
self.B = config.get('B', 250)
# Update ranges
self.R_high = self.R + 25
self.R_low = self.R - 25
self.G_high = self.G + 20
self.G_low = self.G - 20
self.B_high = self.B + 20
self.B_low = self.B - 20
except Exception as e:
self.set_default_config()
def set_default_config(self):
self.trigger_hotkey = 0x02
self.always_enabled = False
self.trigger_delay = 50
self.base_delay = 0.001
self.pixel_fov = 3
self.humanization = False
self.sticky_aim = False
self.current_color = "PURPLE"
self.R, self.G, self.B = 250, 100, 250
self.save_config()
def update_grab_zone(self):
self.grab_zone = calculate_grab_zone(self.pixel_fov)
def initialize(self):
if not self.initialized:
self.sct = dxcam.create(output_color='BGRA', output_idx=0)
self.initialized = True
self.start_main_thread()
def start_main_thread(self):
self.main_thread = threading.Thread(target=self.main_loop)
self.main_thread.daemon = True
self.main_thread.start()
def main_loop(self):
while not self.exit_program:
if not self.paused:
if self.always_enabled or win32api.GetAsyncKeyState(self.trigger_hotkey) < 0:
self.triggerbot = True
self.scan_and_shoot()
else:
self.triggerbot = False
time.sleep(0.001)
def scan_and_shoot(self):
if self.paused:
return
try:
img = np.array(self.sct.grab(self.grab_zone))
if img is None or img.size == 0:
self.sct = dxcam.create(output_color='BGRA', output_idx=0)
return
try:
pixels = img.reshape(-1, 4)
color_mask = (
(pixels[:, 2] > self.R_low) & (pixels[:, 2] < self.R_high) &
(pixels[:, 1] > self.G_low) & (pixels[:, 1] < self.G_high) &
(pixels[:, 0] > self.B_low) & (pixels[:, 0] < self.B_high)
)
if pixels[color_mask].size > 0:
matching_pixels = pixels[color_mask]
self.shoot()
except ValueError as e:
return
except Exception as e:
self.sct = dxcam.create(output_color='BGRA', output_idx=0)
return
@staticmethod
def key_worker(pipe):
# Direct import for faster access
keybd_event = windll.user32.keybd_event
KEY_K = 0x4B # Virtual key code for 'K'
# Set process priority
import win32api
import win32process
import win32con
pid = win32api.GetCurrentProcessId()
handle = win32api.OpenProcess(win32con.PROCESS_ALL_ACCESS, True, pid)
win32process.SetPriorityClass(handle, win32process.HIGH_PRIORITY_CLASS)
# Pre-define sleep time
SLEEP_TIME = 0.001
while True:
try:
# Check pipe without blocking
while pipe.poll():
key = pipe.recv()
if key == 'exit':
return
if key == 'shoot':
# Simulate key press with delay
keybd_event(KEY_K, 0, 0, 0) # Key down
time.sleep(0.01) # Adjust delay as needed
keybd_event(KEY_K, 0, 2, 0) # Key up
# Micro sleep to prevent CPU overload
time.sleep(SLEEP_TIME)
except Exception as e:
print(f"Error in key worker: {e}")
break
def shoot(self):
# Simple delay before shooting
delay = self.base_delay + (self.trigger_delay / 1000.0)
time.sleep(delay)
# Direct shoot command
self.parent_conn.send('shoot')
def save_config(self):
config = {
"trigger_hotkey": hex(self.trigger_hotkey),
"always_enabled": self.always_enabled,
"trigger_delay": self.trigger_delay,
"base_delay": float(self.base_delay),
"pixel_fov": self.pixel_fov,
"auto_counter_strafe": self.auto_counter_strafe,
"humanization": self.humanization,
"sticky_aim": self.sticky_aim,
"current_color": self.current_color,
"R": self.R,
"G": self.G,
"B": self.B
}
with open('config.json', 'w') as json_file:
json.dump(config, json_file, indent=4)
def cleanup(self):
self.exit_program = True
if hasattr(self, 'key_process'):
try:
self.parent_conn.send('exit')
time.sleep(0.1)
self.parent_conn.close()
self.child_conn.close()
self.key_process.join(timeout=1.0)
if self.key_process.is_alive():
self.key_process.terminate()
except:
pass
if self.sticky_aim_thread:
self.sticky_aim_stop_event.set()
self.sticky_aim_thread.join()
def set_always_enabled(self, enabled):
self.always_enabled = enabled
4. Bind the in-game shoot key to «K». If uncomfortable, you can change it in the code.
5. Launch via terminal:
py gui.py
Important!
- Ban risk always exists, even with external hacks. So don’t use cheats on main accounts.
- The project is free, so if you paid for it, you got scammed. Check the GUI and what you’re buying. It might just be repackaged open-source.
- There’s a bug with CPU load. On weak PCs, usage can hit 80–90%. The author is trying to optimize the code, so stay tuned for updates — I’ll post them immediately in this thread.
Frequently asked questions
Why isn’t the cheat shooting?
Check color and FOV settings. If enemies are in camouflage, the bot might not see them.
Why publish cheat source codes?
The author ditched the project, but the code works. You can use it as a base for your experiments.
Where to download a Valorant cheat?
The sources are freely available above under the hide.
Conclusion
I’ve posted colorbots before, and this triggerbot is a great example of how even a free Valorant cheat can farm decently. The undeniable plus of all hacks I’ve shared is the open code. You can test parameters, add Vanguard bypasses, or improve FPS optimization. Use only on accounts you don’t care about. And remember — if someone asks money for these sources, they’re scammers.Reminder: you can download free Valorant 2025 cheats in this section, and all updates for this specific hack will be posted in this thread.