- Status
- Offline
- Joined
- Jul 18, 2023
- Messages
- 379
- Reaction score
- 2
Whether you're a seasoned player or a complete novice just embarking on your adventure in the blocky world of Minecraft, fear not, because our carefully curated selection of mods and textures has something special in store for everyone. Prepare to witness the wonders of this pixelated universe like never before, as we take your gaming experience to new heights with our vast array of enhancements.
For the seasoned veterans, our powerful mods will open up a world of endless possibilities, adding depth and complexity to the game you know and love. Unleash your creativity and explore new gameplay mechanics, taking your skills to the next level as you conquer new challenges.
For the seasoned veterans, our powerful mods will open up a world of endless possibilities, adding depth and complexity to the game you know and love. Unleash your creativity and explore new gameplay mechanics, taking your skills to the next level as you conquer new challenges.
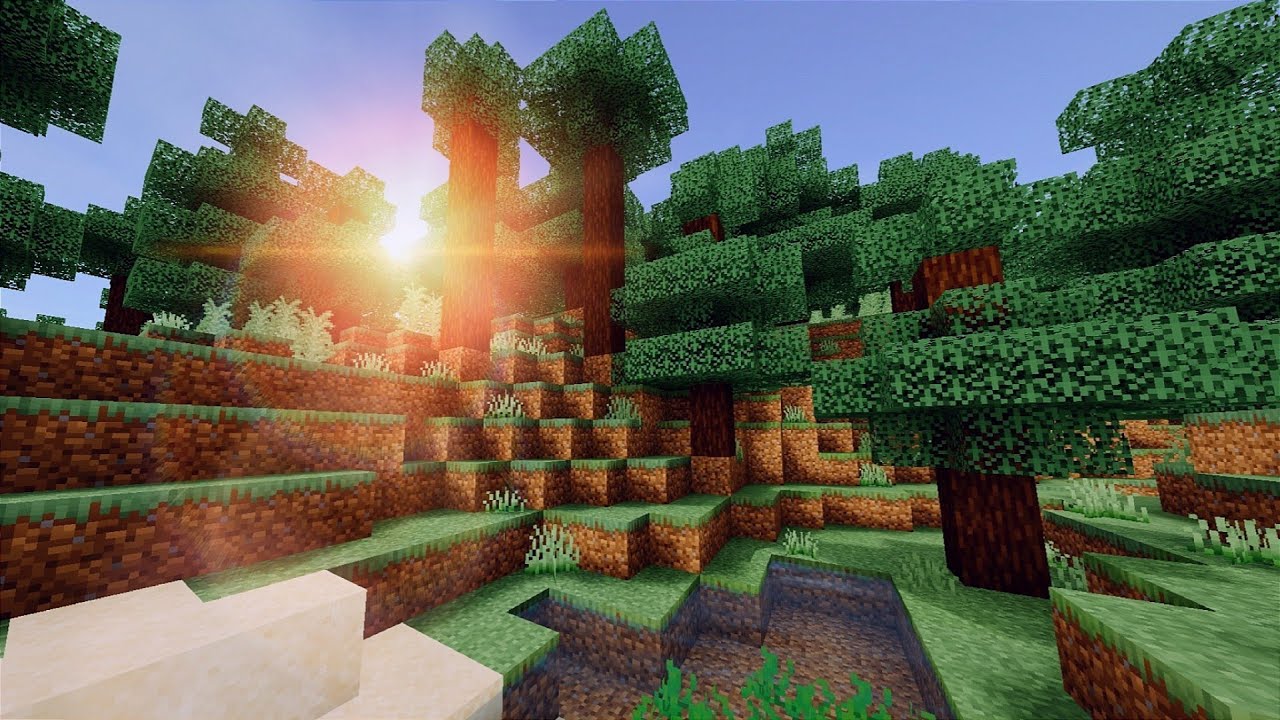
TPAura:
Code:
@ModuleAnnotation(name = "TPAura", type = Type.COMBAT)
public class TPAura extends Module {
public Path path;
public SliderSetting range;
public SliderSetting step;
public BooleanSetting crits;
public BooleanSetting render;
public BooleanSetting swap;
public BooleanSetting rotate;
public static EntityLivingBase entity;
public EventListener<EventMotion> e;
public EventListener<EventDraw> draw;
public TPAura() {
this.range = new SliderSetting("Range", 15.0F, 10.0F, 100.0F, 1.0F);
this.step = new SliderSetting("Step", 5.0F, 2.0F, 50.0F, 1.0F);
this.crits = new BooleanSetting("Criticals", true);
this.render = new BooleanSetting("Visual", true);
this.swap = new BooleanSetting("Swap", false);
this.rotate = new BooleanSetting("Rotate", true);
this.e = (e -> {
setDisplayName(String.valueOf(this.range.get()));
entity = (EntityLivingBase)getNearestEntity();
if (entity == null) {
this.path = null;
return;
}
this.path = new Path(mc.player.getPositionVector(), entity.getPositionVector());
this.path.calculatePath(this.step.get());
if (this.rotate.get()) {
Vec2f rotations = getRotationToEntity((Entity)entity);
e.setYaw(rotations.x);
e.setPitch(rotations.y);
}
if (mc.player.getCooledAttackStrength(1.0F) == 1.0F) {
if (this.crits.get())
mc.player.connection.sendPacket((Packet)new CPacketPlayer.Position(mc.player.posX, mc.player.posY + 0.01D, mc.player.posZ, false));
for (Vec3d vec : this.path.getPath())
mc.player.connection.sendPacket((Packet)new CPacketPlayer.PositionRotation(vec.x, vec.y, vec.z, mc.player.rotationYaw, mc.player.rotationPitch, false));
mc.player.connection.sendPacket((Packet)new CPacketUseEntity((Entity)entity));
mc.player.resetCooldown();
if (this.swap.get())
mc.player.swingArm(EnumHand.MAIN_HAND);
Collections.reverse(this.path.getPath());
for (Vec3d vec : this.path.getPath())
mc.player.connection.sendPacket((Packet)new CPacketPlayer.PositionRotation(vec.x, vec.y, vec.z, mc.player.rotationYaw, mc.player.rotationPitch, false));
}
});
this.draw = (e -> {
if (e.type == EventDraw.RenderType.RENDER) {
if (this.path == null || !this.render.get())
return;
for (Vec3d entity : this.path.getPath()) {
if (mc.player.getPositionVector().distanceTo(entity) < 2.0D)
continue;
double x = entity.x - (mc.getRenderManager()).renderPosX;
double y = entity.y - (mc.getRenderManager()).renderPosY;
double z = entity.z - (mc.getRenderManager()).renderPosZ;
GL11.glPushMatrix();
mc.entityRenderer.setupCameraTransform(e.partialTicks, 2);
GL11.glDisable(3008);
GL11.glBlendFunc(770, 771);
GL11.glEnable(3042);
GL11.glDisable(3553);
GL11.glDepthMask(false);
GlStateManager.color(1.0F, 1.0F, 1.0F, 0.05F);
Render<Entity> render = null;
try {
render = mc.getRenderManager().getEntityRenderObject((Entity)mc.player);
} catch (Exception e1) {
e1.printStackTrace();
}
if (render != null)
((RenderLivingBase)RenderLivingBase.class.cast(render)).doRenderT((EntityLivingBase)mc.player, x, y, z, 1.0F, mc.getRenderPartialTicks());
GL11.glEnable(3553);
GL11.glDepthMask(true);
GL11.glDisable(3042);
GL11.glEnable(3008);
mc.entityRenderer.setupCameraTransform(e.partialTicks, 0);
GlStateManager.resetColor();
GL11.glPopMatrix();
}
}
});
}
public void onDisable() {
super.onDisable();
entity = null;
}
public Vec2f getRotationToEntity(Entity entity) {
Vec3d eyesPos = new Vec3d(mc.player.posX, mc.player.posY + mc.player.getEyeHeight(), mc.player.posZ);
Vec3d entityPos = new Vec3d(entity.posX, entity.posY + entity.getEyeHeight(), entity.posZ);
double diffX = entityPos.x - eyesPos.x;
double diffY = entityPos.y - eyesPos.y;
double diffZ = entityPos.z - eyesPos.z;
double dist = Math.sqrt(diffX * diffX + diffZ * diffZ);
float yaw = (float)Math.toDegrees(Math.atan2(diffZ, diffX)) - 90.0F;
float pitch = (float)-Math.toDegrees(Math.atan2(diffY, dist));
return new Vec2f(yaw, pitch);
}
public Entity getNearestEntity() {
EntityPlayer entityPlayer;
Entity nearest = null;
double distance = this.range.get();
for (EntityPlayer entity : mc.world.playerEntities) {
if (entity == mc.player)
continue;
if (entity.isBot)
continue;
double d = mc.player.getDistanceToEntity((Entity)entity);
if (nearest == null && d < distance) {
entityPlayer = entity;
distance = d;
}
}
return (Entity)entityPlayer;
}
}